Automate Data Import from Google Sheets to Google Ads
Updated
by Raquel Bartolome
These examples assume that you have set up your Google Ads API client library and authenticated correctly. You need to replace placeholder values with actual IDs and paths. Using the methods below, you can successfully upload custom audiences from Google Sheets to Google Ads.
Ensure that your data is hashed if required before uploading to Google Ads. Google Ads may take some time to process and match your customer data.
1. Google Ads Scripts: Create a script that accesses your Google Sheet, reads the data, and uploads it to Google Ads.
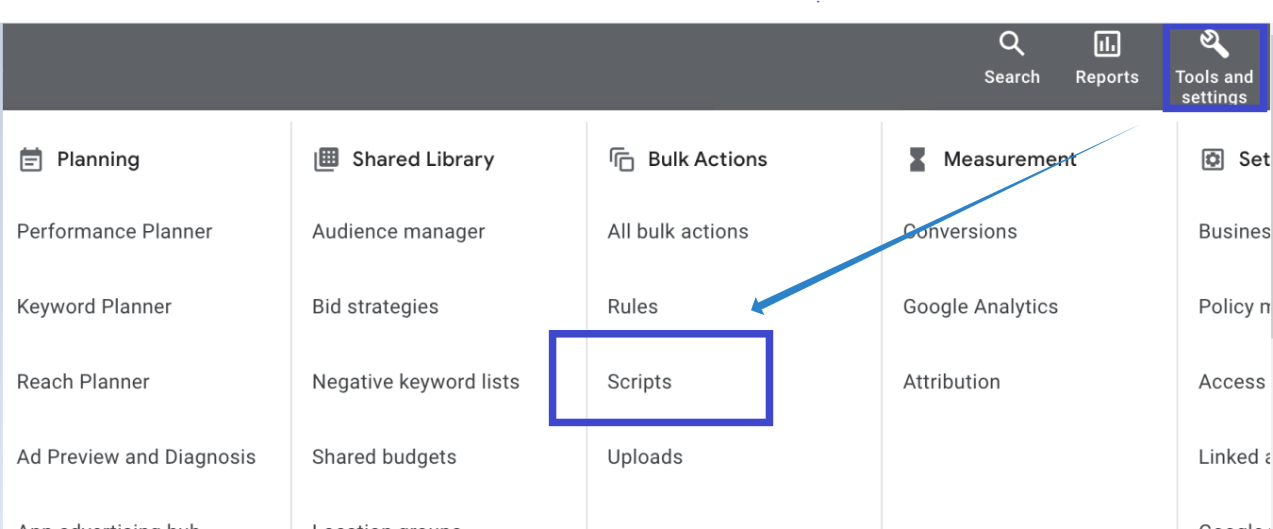
Example: Using Google Ads Scripts (javascript)
function uploadCustomerList() {
var sheetUrl = 'YOUR_GOOGLE_SHEET_URL';
var sheet = SpreadsheetApp.openByUrl(sheetUrl).getSheetByName('Sheet1');
var data = sheet.getDataRange().getValues();
var customerData = data.map(function(row) {
return {
'hashedEmail': Utilities.computeDigest(Utilities.DigestAlgorithm.MD5, row[0]).map(function(e) {
return (e + 256).toString(16).slice(-2);
}).join(''),
// Map other fields as needed
};
});
var audience = AdsApp.createAudienceBuilder()
.withName('Custom Audience')
.withCustomerData(customerData)
.build();
}
2. Google Ads API: Write a script in a language like Python or JavaScript to access your Google Sheet via the Google Sheets API, process the data, and upload it to Google Ads using the Google Ads API.
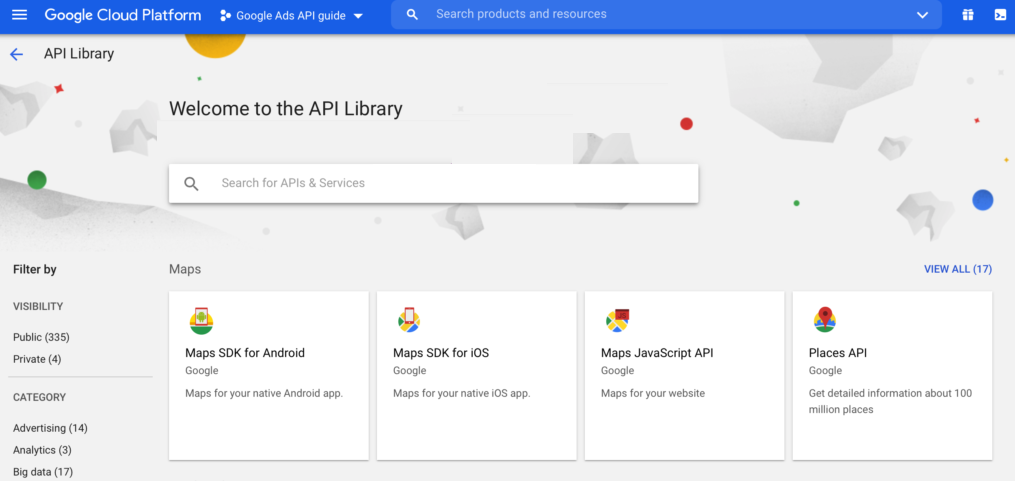
Example: Using Google Ads API (python)
from google.oauth2 import service_account
import googleapiclient.discovery
import csv
# Google Sheets API setup
SCOPES = ['https://www.googleapis.com/auth/spreadsheets.readonly']
SERVICE_ACCOUNT_FILE = 'path/to/service_account.json'
credentials = service_account.Credentials.from_service_account_file(
SERVICE_ACCOUNT_FILE, scopes=SCOPES)
service = googleapiclient.discovery.build('sheets', 'v4', credentials=credentials)
# The ID and range of the spreadsheet.
SPREADSHEET_ID = 'YOUR_SPREADSHEET_ID'
RANGE_NAME = 'Sheet1!A2:E'
# Call the Sheets API
sheet = service.spreadsheets()
result = sheet.values().get(spreadsheetId=SPREADSHEET_ID,
range=RANGE_NAME).execute()
values = result.get('values', [])
# Process the data and upload to Google Ads using Google Ads API
# Assuming you have set up the Google Ads API client library
from google.ads.google_ads.client import GoogleAdsClient
client = GoogleAdsClient.load_from_storage()
customer_id = 'YOUR_CUSTOMER_ID'
def upload_customer_list(client, customer_id, values):
user_list_service = client.get_service('UserListService')
operations = []
for row in values:
operations.append(
user_list_service.UserListOperation.create({
'name': 'Custom Audience',
'description': 'Customer list',
'crm_based_user_list': {
'upload_key_type': client.enums.CustomerMatchUploadKeyTypeEnum.CONTACT_INFO,
'data_source_type': client.enums.UserListCrmDataSourceTypeEnum.FIRST_PARTY,
'members': [
{
'hashed_email': hash_email(row[0]),
# Include other fields as needed
}
]
}
})
)
user_list_service.mutate_user_lists(customer_id, operations)
def hash_email(email):
import hashlib
return hashlib.sha256(email.encode('utf-8')).hexdigest()
upload_customer_list(client, customer_id, values)